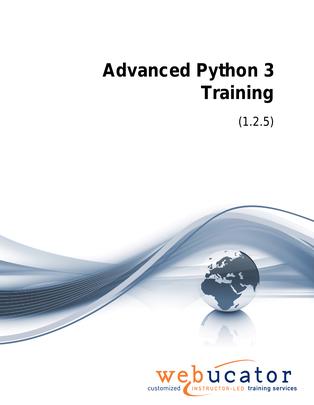
Advanced Python Courseware (PYT238)
This Advanced Python course delves deep into Python concepts to enhance your skills and knowledge of the language. It covers topics such as lambda functions, advanced list comprehensions, and the Collections module. The course also explores regular expressions, working with data using SQLite, CSV, JSON, and web scraping, as well as testing and debugging techniques. Furthermore, it emphasizes object-oriented programming with classes and objects, inheritance, properties, static and class methods, and understanding decorators. Each section includes hands-on exercises to provide practical experience in applying these advanced Python concepts.
This is the updated version of 55285AC from the retired Microsoft Courseware Marketplace.
Benefits
- Comprehensive Coverage: The course covers a wide range of advanced Python topics, ensuring that you have a deep understanding of some of the advanced features of the Python language.
- Practical Examples: The course includes numerous exercises and examples to illustrate advanced concepts, giving you the opportunity to apply your knowledge and skills in real-world scenarios.
- Hands-on Learning: Throughout the course, you will work on practical exercises that reinforce your understanding of the material and build your confidence in using advanced Python techniques.
- Engaging Content: The courseware is designed to be engaging and easy to follow, helping you to stay motivated and focused on learning.
- Experienced Authors: The course is created by expert Python developers with extensive knowledge of advanced Python concepts, ensuring that the material is accurate, relevant, and up-to-date.
Full Lab Environment Add-On
Enhance and simplify your classes by providing an unparalleled learning platform that requires no setup. Your trainers and students can dive straight into a fully-prepared lab environment with just a click. This seamless integration means no time wasted on installations or configurations, allowing trainers and students to focus solely on the task at hand. The lab comes pre-loaded with all the necessary tools and resources, ensuring a smooth, hassle-free learning experience.
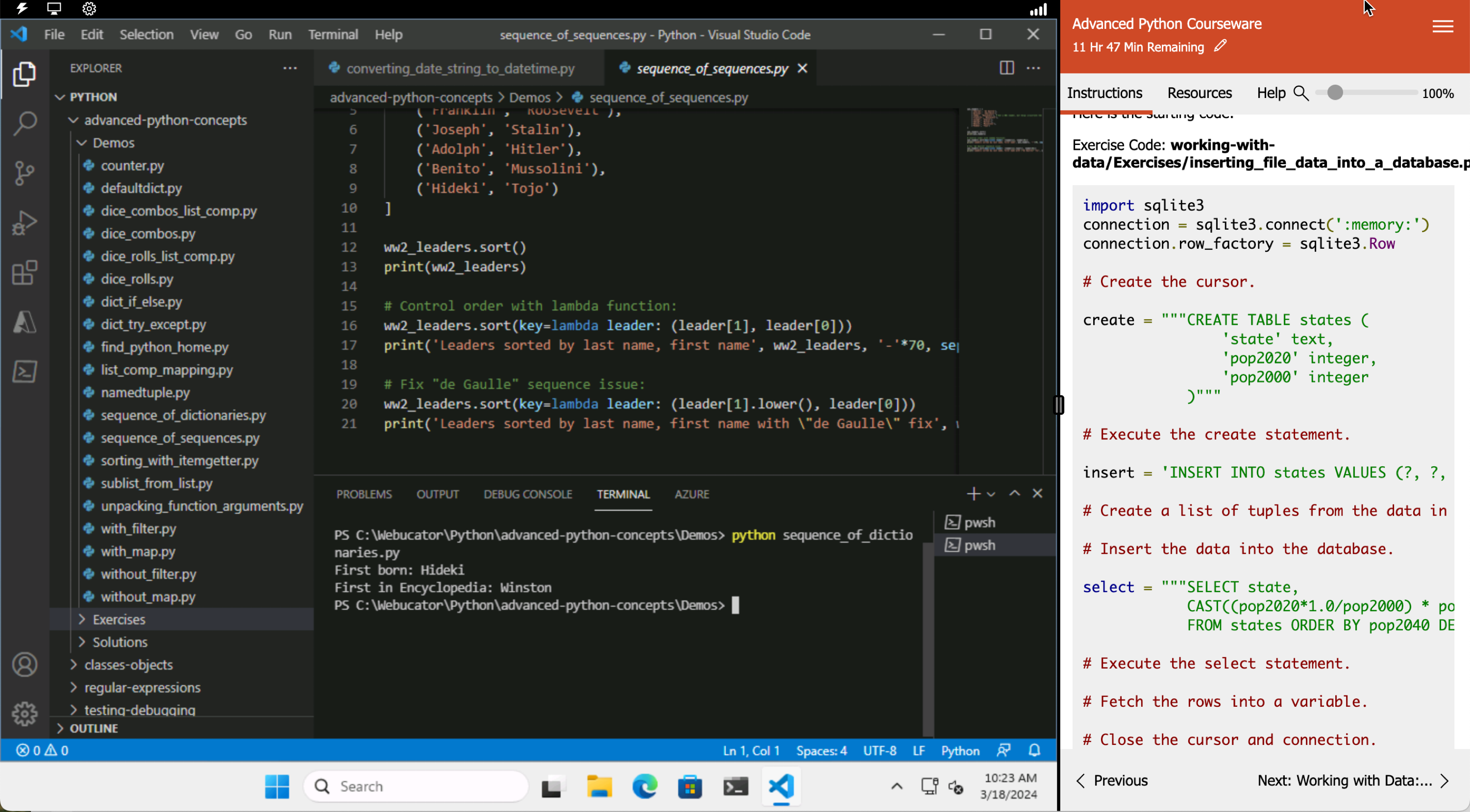
Outline
- Advanced Python Concepts
- Lambda Functions
- Advanced List Comprehensions
- Rolling Five Dice (Exercise)
- Collections Module
- Creating a
defaultdict
(Exercise) - Counters
- Creating a Counter (Exercise)
- Mapping and Filtering
- Mutable and Immutable Built-in Objects
- Sorting
- Converting
list.sort()
tosorted(iterable)
(Exercise) - Sorting Sequences of Sequences
- Creating a Dictionary from Two Sequences
- Unpacking Sequences in Function Calls
- Converting a String to a datetime.date Object (Exercise)
- Modules and Packages
- Regular Expressions
- Regular Expression Tester
- Regular Expression Syntax
- Python’s Handling of Regular Expressions
- Green Glass Door (Exercise)
- Working with Data
- Virtual Environment
- Relational Databases
- Passing Parameters
- SQLite
- Querying a SQLite Database (Exercise)
- SQLite Database in Memory
- Inserting File Data into a Database (Exercise)
- Drivers for Other Databases
- CSV
- Finding Data in a CSV File (Exercise)
- Creating a New CSV File
- Creating a CSV with
DictWriter
(Exercise) - Getting Data from the Web
- HTML Scraping (Exercise)
- XML
- JSON
- JSON Home Runs (Exercise)
- Testing and Debugging
- Testing for Performance
- Comparing Times to Execute (Exercise)
- The
unittest
Module - Fixing Functions (Exercise)
- Special
unittest.TestCase
Methods
- Classes and Objects
- Attributes
- Behaviors
- Classes vs. Objects
- Attributes and Methods
- Adding a
roll()
Method to Die (Exercise) - Private Attributes
- Properties
- Properties (Exercise)
- Objects that Track their Own History
- Documenting Classes
- Documenting the Die Class (Exercise)
- Inheritance
- Extending the Die Class (Exercise)
- Extending a Class Method
- Extending the
roll()
Method (Exercise) - Static Methods
- Class Attributes and Methods
- Abstract Classes and Methods
- Understanding Decorators
Required Prerequisites
- Basic Python programming experience. In particular, you should be very comfortable with:
- Working with strings.
- Working with lists, tuples and dictionaries.
- Loops and conditionals.
- Writing your own functions.
Useful Prerequisites
- Some exposure to HTML, XML, JSON, and SQL.
License
Length: 2
days | $90.00 per copy
Labs: Add-on available | $25.49 per lab
View Lab Details
What is Included?
- Student Manual
- Student Class Files
- Labs (optional add-on)