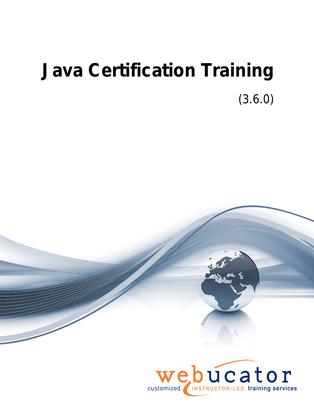
Java Certification Courseware (JVA103)
The Java Certification course offers a comprehensive and in-depth curriculum to help students master Java programming. It covers topics ranging from the basics of Java and object-oriented programming to more advanced topics such as inheritance, interfaces, and exceptions. Students will also dive into collections, inner classes, and streams, as well as explore advanced topics such as concurrency, JDBC, localization, security, and modules. This course is designed to equip students with the knowledge and skills required to excel in Java programming and achieve Java certification.
This course helps prepare students for the Java SE 17 Developer exam, which is the only exam needed to become an Oracle Certified Professional: Java SE 17 Developer.
Benefits
- Comprehensive Coverage: The Java Certification course covers all essential topics and skills needed to excel in Java programming, providing a complete learning experience for students.
- Practical Examples: The courseware includes numerous practical examples and exercises that help students understand concepts better and gain hands-on experience in solving real-world problems.
- Hands-on Learning: The course encourages students to learn through hands-on activities, such as coding exercises and project-based assignments, ensuring that they develop a deep understanding of the concepts and can apply them in real-life scenarios.
- Engaging Content: The course material is designed to be engaging and interactive, making the learning experience enjoyable and effective for both trainers and students.
- Experienced Authors: The courseware is designed and curated by industry experts with years of experience in Java programming and education, ensuring that the content is accurate, relevant, and up-to-date.
Outline
- Java Introduction
- The Java Environment - Overview
- Writing a Java Program
- Obtaining The Java Environment
- Setting Up Your Java Environment
- Creating a Class that Can Run as a Program
- Useful Stuff Necessary to Go Further
- Using an Integrated Development Environment
- Running a Simple Java Program
- Using the Java Documentation
- Java Basics
- Basic Java Syntax
- Variables
- Data
- Constants and the final Keyword
- Mathematics in Java
- Creating and Using Methods
- Variable Scope
- Method Exercise
- Java Objects
- Objects
- Object-oriented Languages
- Object Definition
- References
- Defining a Class
- More on Access Terms
- Adding Data Members to a Class
- Standard Practices for Fields and Methods
- Java Beans
- Bean Properties
- Payroll01: Creating an Employee Class
- Constructors
- Instantiating Objects Revisited
- Important Note on Constructors
- Payroll02: Adding an Employee Constructor
- Method Overloading
- Payroll03: Overloading Employee Constructors
- The this Keyword
- Using this to Call Another Constructor
- Payroll04: Using the this Reference
- static Elements
- The main Method
- Payroll05: A static Field in Employee
- Garbage Collection
- Java Packages
- Compiling with Packages
- Working with Packages
- Payroll06: Creating an employees Package
- Variable Argument Lists (varargs)
- Payroll07: Using KeyboardReader in Payroll
- Creating Documentation Comments and Using javadoc
- Payroll08: Creating and Using javadoc Comments
- Primitives and Wrapper Classes
- Encapsulation
- String, StringBuffer, and StringBuilder
- Compiling and Executing with Packages
- Object-oriented Programs
- Comparisons and Flow Control Structures
- Boolean-valued Expressions
- Comparison Operators
- Comparing Objects
- Conditional Expression Examples
- Complex boolean Expressions
- Simple Branching
- The if Statement
- if Statement Examples
- Payroll-Control01: Modified Payroll
- Two Mutually Exclusive Branches
- Comparing a Number of Mutually Exclusive Options - The switch Statement
- Comparing Two Objects
- Conditional Expression
- Payroll-Control02: Payroll with a Loop
- Additional Loop Control: break and continue
- Continuing a Loop
- Classpath, Code Libraries, and Jar Files
- Creating and Using an External Library
- Compiling to a Different Directory
- Conditionals and Loops
- Game02: A Revised Guessing Game
- Game01: A Guessing Game
- Game03: Multiple Levels
- Game04: Guessing Game with a Loop
- Arrays
- Defining and Declaring Arrays
- Instantiating Arrays
- Initializing Arrays
- Working with Arrays
- Enhanced for Loops - the For-Each Loop
- Array Variables
- Copying Arrays
- Using the args Array
- Arrays of Objects
- Payroll-Arrays01: An Array of Employees
- Multi-Dimensional Arrays
- Multidimensional Arrays in Memory
- Example - Printing a Picture
- Typecasting with Arrays of Primitives
- Java Arrays
- Game-Arrays01: A Guessing Game with Random Messages
- Inheritance
- Inheritance
- Payroll with Inheritance
- Polymorphism
- Creating a Subclass
- Inheritance and Access
- Inheritance and Constructors - the super Keyword
- Example - Factoring Person Out of Employee
- Payroll-Inheritance01: Adding Types of Employees
- Inheritance and Default Superclass Constructors
- Typecasting with Object References
- More on Overriding
- Payroll-Inheritance02: Using the Employee Subclasses
- Other Inheritance-related Keywords
- Payroll-Inheritance03: Making Our Base Classes Abstract
- Methods Inherited from Object
- Checking an Object’s Type: Using instanceof
- The Instantiation Process at Runtime
- Inheritance Example - A Derived Class
- Inheritance Examples
- Derived Class Objects
- Derived Class Methods that Override Base Class Methods
- Object Typecasting Example
- Typecasting with Arrays of Objects
- Interfaces
- Interfaces
- Creating an Interface Definition
- Implementing Interfaces
- Reference Variables and Interfaces
- Interfaces and Inheritance
- Exercise: Payroll-Interfaces01
- Some Uses for Interfaces
- Annotations
- Using Annotations
- Annotation Details
- Exceptions
- Exceptions
- Handling Exceptions
- Exception Objects
- Attempting Risky Code - try and catch
- Guaranteeing Execution of Code - The finally Block
- Letting an Exception be Thrown to the Method Caller
- Throwing an Exception
- Payroll-Exceptions01: Handling NumberFormatException in Payroll
- Exceptions and Inheritance
- Creating and Using Your Own Exception Classes
- Payroll-Exceptions02
- Rethrowing Exceptions
- Initializer Blocks
- Logging
- Log Properties
- Assertions
- Collections
- Collections
- Using the Collection Classes
- Using the Iterator Interface
- Creating Collectible Classes
- Generics
- Bounded Types
- Extending Generic Classes and Implementing Generic Interfaces
- Generic Methods
- Variations on Generics - Wildcards
- Type Erasure
- Multiple-bounded Type Parameters
- Payroll-Collections01: Payroll Using Generics
- Working with Streams and Lambda expressions
- Working with Streams and Lambda expressions
- Inner Classes
- Inner Classes, aka Nested Classes
- Inner Class Syntax
- Instantiating an Inner Class Instance from within the Enclosing Class
- Inner Classes Referenced from Outside the Enclosing Class
- Referencing the Outer Class Instance from the Inner Class Code
- Better Practices for Working with Inner Classes
- Enums
- Inner Classes
- Method Inner Classes
- Anonymous Inner Classes
- Streams
- Introducing Streams
- Input Stream Classes
- Output Stream Classes
- Using System.in
- File and Directory Information
- Creating a Directory Listing
- Files and Streams
- Creating a File Copying Program
- Dealing with Binary Data
- Java Primitives as Binary Data
- Sending and Receiving Objects - Serialization
- Payroll-Streams01
- Properties
- Java NIO
- Advanced Topics
- Concurrency
- JDBC
- Localization
- Security
- Modules
Required Prerequisites
None
Useful Prerequisites
- Object-oriented Programming Experience in a language such as C++ or C#.
License
Length: 5
days | $175.00 per copy
What is Included?
- Student Manual
- Student Class Files