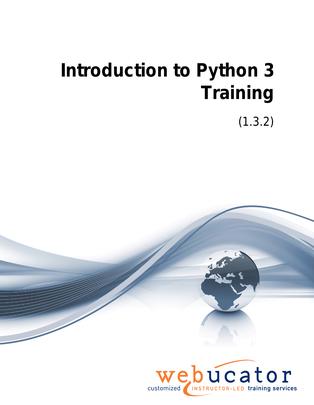
Introduction to Python Courseware (PYT138)
This Introduction to Python course provides a comprehensive foundation in Python programming, starting with Python basics such as running Python scripts, understanding literals, comments, data types, and working with variables. The course then progresses to functions and modules, arithmetic operations, and Python string manipulation. You'll also learn about iterables, including sequences, dictionaries, and sets, as well as virtual environments, packages, and pip. The course covers flow control, exception handling, Python dates and times, and file processing. Finally, it introduces you to PEP8 and Pylint for code quality and style. Each section includes exercises to help you practice your newfound skills and solidify your understanding of Python programming concepts.
This is the updated version of 55284AC from the retired Microsoft Courseware Marketplace.
Benefits
- Easy-to-follow curriculum: The course is structured to gradually introduce Python concepts, starting with the basics and progressing to more advanced topics. This step-by-step approach allows students to build their skills and confidence at a comfortable pace.
- Engaging, hands-on learning: Our course emphasizes practical coding exercises and real-life examples, enabling students to apply their newfound Python knowledge to actual programming challenges. This hands-on approach helps solidify understanding and retention.
- Expert authors: The course was written and is maintained by experienced instructors with a deep understanding of Python and a passion for teaching. Their expertise ensures that the course provides clear explanations, practical exercises, and engaging content.
- Strong foundation for future learning: By covering fundamental Python concepts, the course lays the groundwork for students to pursue more advanced programming topics, setting them on a path to becoming proficient Python developers.
Full Lab Environment Add-On
Enhance and simplify your classes by providing an unparalleled learning platform that requires no setup. Your trainers and students can dive straight into a fully-prepared lab environment with just a click. This seamless integration means no time wasted on installations or configurations, allowing trainers and students to focus solely on the task at hand. The lab comes pre-loaded with all the necessary tools and resources, ensuring a smooth, hassle-free learning experience.
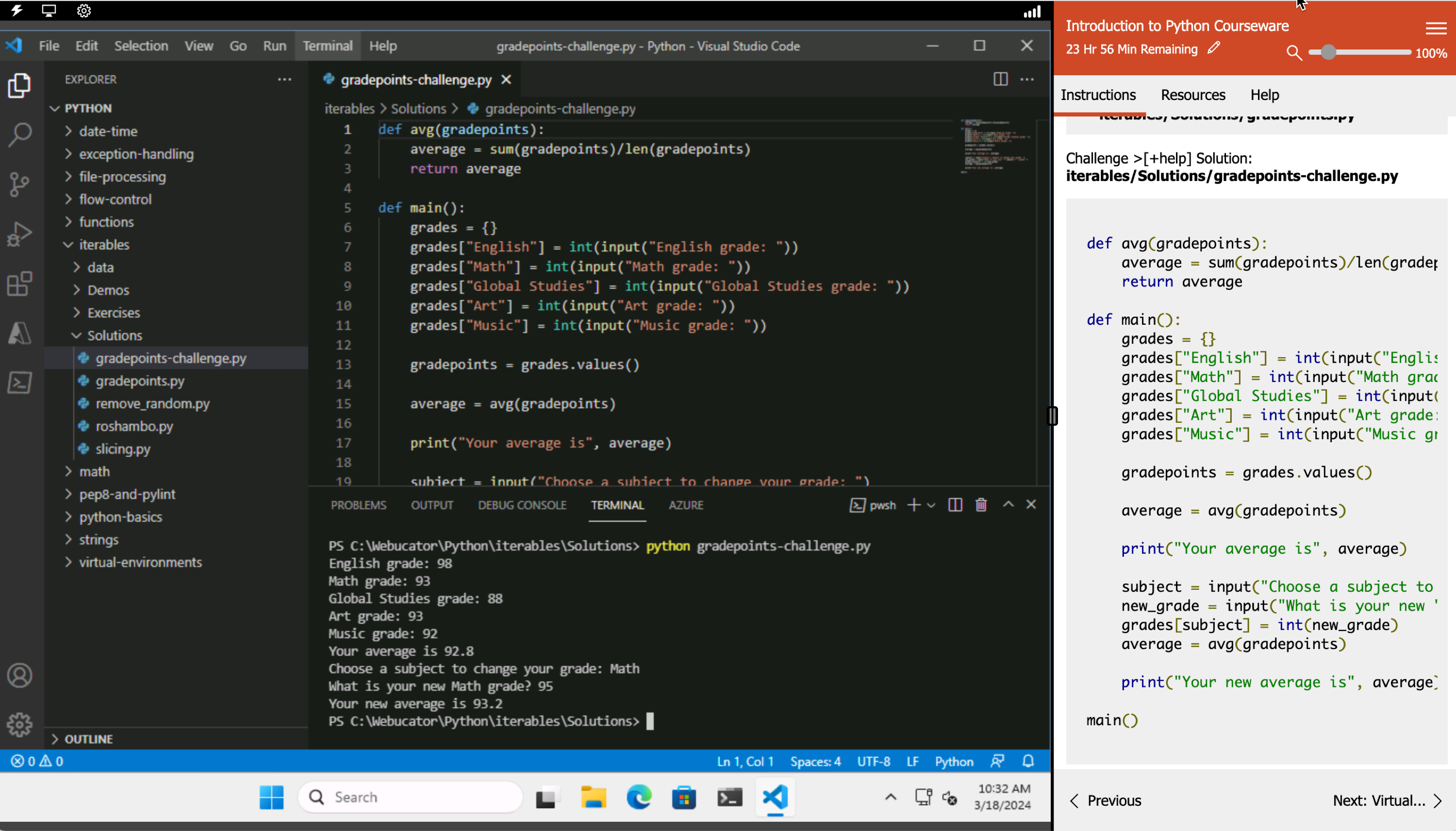
Outline
- Python Basics
- Getting Familiar with the Terminal
- Running Python
- Running a Python File
- Hello, world! (Exercise)
- Literals, Comments, and Data Types
- Exploring Types (Exercise)
- Variables
- A Simple Python Script (Exercise)
- Constants and Deleting Variables
- Writing a Python Module
print()
Function- Collecting User Input
- Hello, You! (Exercise)
- Reading from and Writing to Files
- Working with Files (Exercise)
- Functions and Modules
- Defining Functions
- Variable Scope
- Global Variables
- Function Parameters
- A Function with Parameters (Exercise)
- Default Values
- Parameters with Default Values (Exercise)
- Returning Values
- Importing Modules
- Methods vs. Functions
- Math
- Arithmetic Operators
- Floor and Modulus (Exercise)
- Assignment Operators
- Precedence of Operations
- Built-in Math Functions
- The
math
Module - The
random
Module - How Many Pizzas Do We Need? (Exercise)
- Dice Rolling (Exercise)
- Python Strings
- Quotation Marks and Special Characters
- String Indexing
- Indexing Strings (Exercise)
- Slicing Strings
- Slicing Strings (Exercise)
- Concatenation and Repetition
- Repetition (Exercise)
- Combining Concatenation and Repetition
- Python Strings are Immutable
- Common String Methods
- String Formatting
- Playing with Formatting (Exercise)
- Formatted String Literals (f-strings)
- Built-in String Functions
- Outputting Tab-delimited Text (Exercise)
- Iterables: Sequences, Dictionaries, and Sets
- Definitions
- Sequences
- Lists
- Sequences and Random
- Remove and Return Random Element (Exercise)
- Tuples
- Ranges
- Converting Sequences to Lists
- Indexing
- Simple Rock, Paper, Scissors Game (Exercise)
- Slicing
- Slicing Sequences (Exercise)
min()
,max()
, andsum()
- Converting between Sequences and Strings
- Unpacking Sequences
- Dictionaries
- The
len()
Function - Creating a Dictionary from User Input (Exercise)
- Sets
*args
and**kwargs
- Virtual Environments, Packages, and
pip
- Creating, Activating, Deactivating, and Deleting a Virtual Environment (Exercise)
- Packages with
pip
- Working with a Virtual Environment (Exercise)
- Flow Control
- Conditional Statements
- Compound Conditions
- The
is
andis not
Operators all()
andany()
and the Ternary Operator- In Between
- Loops in Python
- All True and Any True (Exercise)
break
andcontinue
- Looping through Lines in a File
- Word Guessing Game (Exercise)
- The
else
Clause in Loops for…else
(Exercise)- The
enumerate()
Function - Generators
- List Comprehensions
- Exception Handling
- Exception Basics
- Generic Exceptions
- Raising Exceptions (Exercise)
- The
else
andfinally
Clauses - Using Exceptions for Flow Control
- Running Sum (Exercise)
- Raising Your Own Exceptions
- Python Dates and Times
- Understanding Time
- The time Module
- Time Structures
- Times as Strings
- Time and Formatted Strings
- Pausing Execution with
time.sleep()
- The
datetime
Module datetime.datetime
Objects- What Color Pants Should I Wear? (Exercise)
datetime.timedelta
Objects- Report on Departure Times (Exercise)
- File Processing
- Paths
- The
pathlib
Module - Opening Files
- Finding Text in a File (Exercise)
- Writing to Files
- Writing to Files (Exercise)
- List Creator (Exercise)
- Path Methods for Reading and Writing Files
- Making Directories
- Deleting Files and Directories
- Renaming Files and Directories
- The
os
Module - A Better Way to Open Files
- Comparing Lists (Exercise)
- PEP8 and Pylint
- PEP8
- Pylint
Required Prerequisites
None
Useful Prerequisites
- Some programming experience.
License
Length: 4
days | $180.00 per copy
Labs: Add-on available | $50.97 per lab
View Lab Details
What is Included?
- Student Manual
- Student Class Files
- Labs (optional add-on)