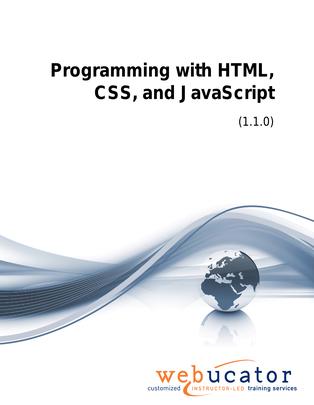
Programming with HTML, CSS, and JavaScript Courseware (HCJ501)
In this fast-paced course, you'll learn the fundamentals of client-side web development, starting with the basics of HTML for structuring content. We will cover various HTML elements, attributes, and techniques for building a well-structured web page. Next, you'll dive into CSS to learn about styling your web pages with various properties and selectors, making your content visually appealing. Finally, we will explore JavaScript, a powerful programming language that allows you to add interactivity and dynamic content to your websites. Throughout the course, you'll have the opportunity to work on practical exercises and hands-on examples, solidifying your knowledge and preparing you for real-world web development projects.
This is the updated version of 55320AC from the retired Microsoft Courseware Marketplace.
Benefits
- Comprehensive Coverage: Our courseware covers a wide range of topics, from HTML and CSS fundamentals to JavaScript programming, ensuring a well-rounded understanding of web development.
- Practical Examples: The course is packed with hands-on examples and exercises, allowing you to apply your knowledge and build confidence in your web development skills.
- Hands-on Learning: We emphasize learning by doing, ensuring that you get plenty of opportunities to practice and refine your web development skills throughout the course.
- Engaging Content: Our course content is designed to be engaging and enjoyable, making it easier for you to stay focused and motivated as you learn.
- Experienced Authors: Our courseware is developed by experts in the field of web development, ensuring that you receive high-quality, up-to-date, and relevant training.
PowerPoint Presentation
This course includes a PowerPoint presentation that maps to the manual and to the labs:
Full Lab Environment Add-On
Enhance and simplify your classes by providing an unparalleled learning platform that requires no setup. Your trainers and students can dive straight into a fully-prepared lab environment with just a click. This seamless integration means no time wasted on installations or configurations, allowing trainers and students to focus solely on the task at hand. The lab comes pre-loaded with all the necessary tools and resources, ensuring a smooth, hassle-free learning experience.
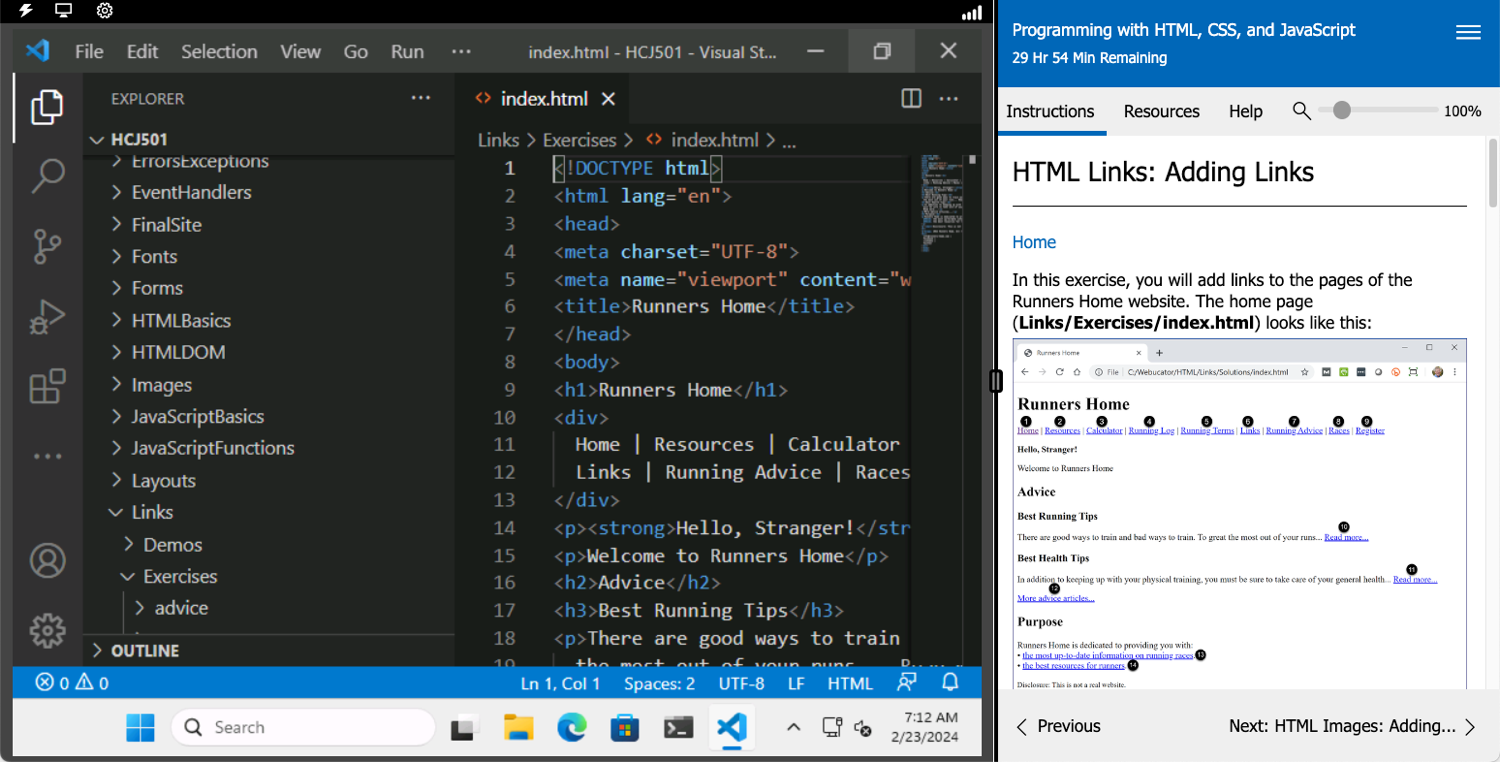
Outline
- A Quick Overview of Web Development
- HTML is Part of a Team
- Client-side Programming
- Server-side Programming
- Introduction to HTML
- A Simple HTML Document (Exercise)
- HTML Elements, Attributes, and Comments
- The HTML Skeleton
- Viewing the Page Source
- Special Characters
- History of HTML
- The lang Attribute
- Paragraphs, Headings, and Text
- Paragraphs
- Heading Levels
- Breaks and Horizontal Rules
- The div Tag
- Creating an HTML Page (Exercise)
- Quoted Text
- Preformatted Text
- Inline Semantic Elements
- Adding Inline Elements (Exercise)
- HTML Links
- Text Links
- Absolute vs. Relative Paths
- Targeting New Tabs
- Email Links
- Adding Links (Exercise)
- Lorem Ipsum
- The title Attribute
- Targeting a Specific Location on the Page
- HTML Images
- Inserting Images
- Image Links
- Adding Images to the Page (Exercise)
- Providing Alternative Images
- HTML Lists
- Unordered Lists
- Ordered Lists
- Definition Lists
- Creating Lists (Exercise)
- Sectioning a Web Page
- Semantic Block-Level Elements
- Articles vs. Sections
- Sectioning the Home Page
- Sectioning Content and Styling
- Sectioning the Pages (Exercise)
- Crash Course in CSS
- Benefits of Cascading Style Sheets
- CSS Rules
- Selectors
- Combinators
- Precedence of Selectors
- How Browsers Style Pages
- CSS Resets
- CSS Normalizers
- External Stylesheets, Embedded Stylesheets, and Inline Styles
- Creating an External Stylesheet (Exercise)
- Creating an Embedded Stylesheet (Exercise)
- Adding Inline Styles (Exercise)
- div and span
- Styling div and span (Exercise)
- Media Types
- Units of Measurement
- Inheritance
- CSS Fonts
- font-family
- @font-face
- font-size
- font-style
- font-variant
- font-weight
- line-height
- font
- Styling Fonts (Exercise)
- Color and Opacity
- About Color and Opacity
- Color and Opacity Values
- color
- opacity
- Adding Color and Opacity to Text (Exercise)
- CSS Text
- letter-spacing
- text-align
- text-decoration
- text-indent
- text-shadow
- text-transform
- white-space
- word-break
- word-spacing
- Text Properties (Exercise)
- JavaScript Basics
- JavaScript vs. EcmaScript
- The HTML DOM
- JavaScript Syntax
- Accessing Elements
- Where Is JavaScript Code Written?
- JavaScript Objects, Methods, and Properties
- Alerts, Writing, and Changing Background Color (Exercise)
- Variables, Arrays, and Operators
- JavaScript Variables
- A Loosely Typed Language
- Google Chrome DevTools
- Storing User-Entered Data
- Using Variables (Exercise)
- Constants
- Arrays
- Working with Arrays (Exercise)
- Associative Arrays
- Playing with Array Methods
- JavaScript Operators
- The Modulus Operator
- Playing with Operators
- The Default Operator
- Working with Operators (Exercise)
- JavaScript Functions
- Global Objects and Functions
- Working with Global Functions (Exercise)
- User-defined Functions
- Writing a JavaScript Function (Exercise)
- Returning Values from Functions
- Built-In JavaScript Objects
- Strings
- Math
- Date
- Helper Functions
- Returning the Day of the Week as a String (Exercise)
- Conditionals and Loops
- Conditionals
- Short-circuiting
- Switch / Case
- Ternary Operator
- Truthy and Falsy
- Conditional Processing (Exercise)
- Loops
- while and do…while Loops
- for Loops
- break and continue
- Working with Loops (Exercise)
- Array: forEach()
- Event Handlers and Listeners
- On-event Handlers
- Using On-event Handlers (Exercise)
- The addEventListener() Method
- Anonymous Functions
- Capturing Key Events
- Adding Event Listeners (Exercise)
- Benefits of Event Listeners
- Timers
- Typing Test (Exercise)
- The HTML Document Object Model
- CSS Selectors
- The innerHTML Property
- Nodes, NodeLists, and HTMLCollections
- Accessing Element Nodes
- Accessing Elements (Exercise)
- Dot Notation and Square Bracket Notation
- Accessing Elements Hierarchically
- Working with Hierarchical Elements (Exercise)
- Accessing Attributes
- Creating New Nodes
- Focusing on a Field
- Shopping List Application
- Logging (Exercise)
- Adding EventListeners (Exercise)
- Adding Items to the List (Exercise)
- Dynamically Adding Remove Buttons to the List Items (Exercise)
- Removing List Items (Exercise)
- Preventing Duplicates and Zero-length Product Names (Exercise)
- Manipulating Tables
- HTML Forms
- How HTML Forms Work
- The form Element
- Form Elements
- Buttons
- Creating a Registration Form (Exercise)
- Checkboxes
- Radio Buttons
- Adding Checkboxes and Radio Buttons (Exercise)
- Fieldsets
- Select Menus
- Textareas
- Adding a Select Menu and a Textarea (Exercise)
- HTML Forms and CSS
- JavaScript Form Validation
- Server-side Form Validation
- HTML Form Validation
- Accessing Form Data
- Form Validation with JavaScript
- Checking the Validity of the Email and URL Fields (Exercise)
- Checking Validity on Input and Submit Events
- Adding Error Messages
- Validating Textareas
- Validating Checkboxes
- Validating Radio Buttons
- Validating Select Menus
- Validating the Ice Cream Order Form (Exercise)
- Giving the User a Chance
- Regular Expressions
- Getting Started
- Regular Expression Syntax
- Backreferences
- Form Validation with Regular Expressions
- Cleaning Up Form Entries
- Cleaning Up Form Entries (Exercise)
- A Slightly More Complex Example
Required Prerequisites
None
License
Length: 5
days | $175.00 per copy
Labs: Add-on available | $63.71 per lab
View Lab Details
What is Included?
- Student Manual
- Student Class Files
- PowerPoint Presentation
- Labs (optional add-on)