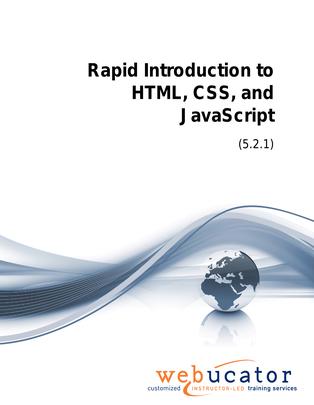
Rapid Introduction to HTML, CSS, and JavaScript Courseware (HCJ101)
In this course, students get a rapid hands-on introduction to client-side programming with HTML, CSS, and JavaScript. The course is meant for students who want to need to get a jump start on all three technologies. Topics covered in the course include an overview of web development, HTML elements and attributes, CSS styling and layout, and JavaScript programming concepts such as variables, arrays, functions, and event handlers.
Students should be prepared to cover a lot of material in a short time and to be ready to spend some time afterward reviewing the content on their own to solidify and build upon the skills taught in class.
Benefits
- Time-efficient learning: This rapid introduction course allows students to quickly acquire essential web development skills, making it perfect for those with limited time or who want to jump-start their learning.
- Comprehensive coverage: The course covers all three core technologies (HTML, CSS, and JavaScript) required for client-side web development, providing a well-rounded understanding of the field.
- Practical skills: Students will gain hands-on experience through various exercises, helping them apply their knowledge to real-world scenarios and develop practical web development skills.
- Dynamic websites: By learning JavaScript, students will be able to create interactive and dynamic websites, improving user experience and engagement.
PowerPoint Presentation
This course includes a PowerPoint presentation that maps to the manual and to the labs:
Outline
- A Quick Overview of Web Development
- Client-side Programming
- HTML
- Cascading Style Sheets
- JavaScript 1
- Ajax
- JavaScript Frameworks
- Server-side Programming
- Java EE
- ASP.NET
- Python
- PHP
- ColdFusion
- Node.js
- Client-side Programming
- Introduction to HTML
- Getting Started
- A Simple HTML Document
- The HTML Skeleton
- The <head> Element
- The <body> Element
- Whitespace
- HTML Elements
- Attributes
- Empty vs. Container Tags
- Blocks and Inline Elements
- Comments
- Special Characters
- History of HTML
- lang Attribute
- Paragraphs, Headings, and Text
- Paragraphs
- Breaks and Horizontal Rules
- Creating an HTML Page
- Quoted Text
- Preformatted Text
- Text-Level Semantic Elements
- HTML Links
- Text Links
- Absolute vs. Relative Paths
- Absolute Paths
- Relative Paths
- Default Pages
- Targeting New Windows
- Email Links
- Adding Links
- Targeting a Specific Location on the Page
- The title Attribute
- HTML Images
- Inserting Images
- Making Images Accessible
- Alternative Text
- Long Descriptions
- Height and Width Attributes
- Image Links
- Adding Images to the Page
- Inserting Images
- HTML Lists
- Unordered Lists
- Nesting Unordered Lists
- Ordered Lists
- Nesting Ordered Lists
- The type Attribute
- The start Attribute
- Definition Lists
- Creating Lists
- Unordered Lists
- Crash Course in CSS
- Benefits of Cascading Style Sheets
- CSS Rules
- CSS Comments
- Selectors
- Type Selectors
- Class Selectors
- ID Selectors
- Attribute Selectors
- The Universal Selector
- Grouping
- Combinators
- Descendant Combinators
- Child Combinators
- General Sibling Combinators
- Adjacent Sibling Combinators
- Precedence of Selectors
- How Browsers Style Pages
- CSS Resets
- CSS Normalizers
- External Stylesheets, Embedded Stylesheets, and Inline Styles
- External Stylesheets
- Embedded Stylesheets
- Inline Styles
- Exercise: Creating an External Stylesheet
- Exercise: Creating an Embedded Stylesheet
- Exercise: Adding Inline Styles
- <div> and <spa>
- Exercise: Divs and Spans
- Media Types
- Units of Measurement
- Absolute vs. Relative Units
- Pixels
- Ems and Rems
- Percentages
- Other Units
- Inheritance
- The inherit Value
- CSS Fonts
- font-family
- Specifying by Font Name
- Specifying Font by Category
- @font-face
- Getting Fonts
- font-size
- Relative font-size Terms
- Best Practices
- font-style
- font-variant
- font-weight
- line-height
- font
- Exercise: Styling Fonts
- font-family
- Color and Opacity
- About Color and Opacity
- Color and Opacity Values
- Color Keywords
- RGB Hexadecimal Notation
- RGB Functional Notation
- HSL Functional Notation
- color
- opacity
- Exercise: Adding Color and Opacity to Text
- CSS Text
- letter-spacing
- text-align
- text-decoration
- text-indent
- text-shadow
- text-transform
- white-space
- word-break
- word-spacing
- Exercise: Text Properties
- JavaScript Basics
- The Name "JavaScript"
- What is ECMAScript?
- The HTML DOM
- JavaScript Syntax
- Accessing Elements
- Dot Notation
- Square Bracket Notation
- Where Is JavaScript Code Written?
- JavaScript Objects, Methods and Properties
- Variables, Arrays, and Operators
- JavaScript Variables
- A Loosely-Typed Language
- Google Chrome DevTools
- Variable Naming
- Storing User-Entered Data
- Constants
- Arrays
- Associative Arrays
- Array Properties and Methods
- Playing with Array Methods
- JavaScript Operators
- The Modulus Operator
- The Default Operator
- JavaScript Variables
- JavaScript Functions
- Global Objects and Functions
- parseFloat(object)
- parseInt(object)
- isNaN(object)
- User-defined Functions
- Function Syntax
- Passing Values to Functions
- Returning Values from Functions
- Global Objects and Functions
- Built-In JavaScript Objects
- String
- Math
- Date
- Helper Functions
- Conditionals and Loops
- Conditionals
- if - else if - else Conditions
- Short-circuiting
- Switch / Case
- Ternary Operator
- Truthy and Falsy
- Loops
- while Loop Syntax
- do while Loop Syntax
- for Loop Syntax
- for of Loop Syntax
- for in Loop Syntax
- break and continue
- Array: forEach()
- Event Handlers and Listeners
- On-Event Handlers
- The getElementById() Method
- The addEventListener() Method
- Capturing Key Events
- Benefits of Event Listeners
- Timers
- On-Event Handlers
Required Prerequisites
- Working with computers regularly
Useful Prerequisites
- Programming Experience
- A basic understanding of how the web works
License
Length: 3
days | $105.00 per copy
What is Included?
- Student Manual
- Student Class Files
- PowerPoint Presentation